In this easy tutorial for beginners, a simple “Hello World” program is created using GTK+ 3 and programmed in the C programming language. Glade 3 is used to create the GUI window, button and text label in this GTK 3 C code hello world tutorial.
The image below shows the windowed Hello World application made in this tutorial. When the button in the window is clicked for the first time, the text “Hello, world!” is displayed in the window. Each time the button is clicked, the total number of button clicks is updated and displayed in the window.
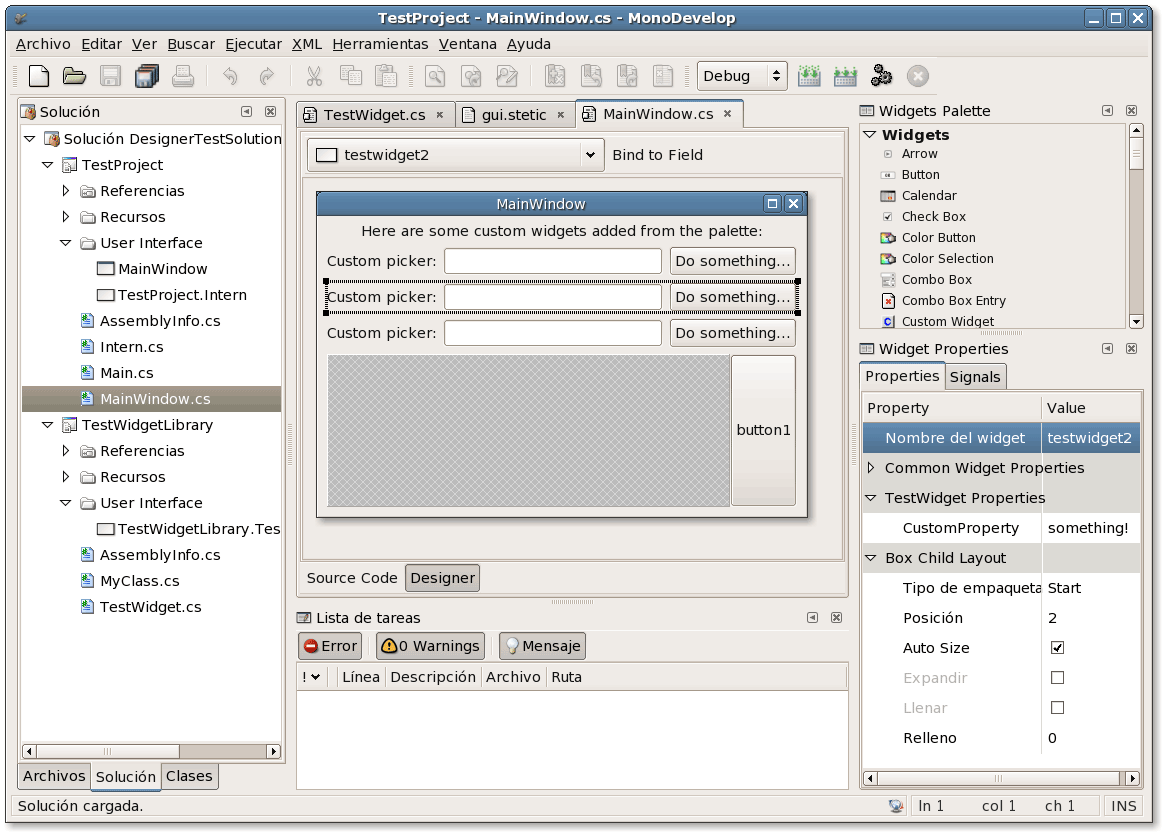
Gtk# is the core of the windowing and widget system (a widget is like a control in SWF). Glade# inherits Gtk# so its a subset of Gtk# and usually compatible, but it automatically layouts out the widgets with an XML based resource file. The XML to layout your GUI can be generated with the Glade tool, a WYSIWYG GUI designer studio. # How to deploy GTK based app on Mac OS X? Many friends ask me to provide the official installer package(.dmg) for Mac OS X, I do want to implement it. But there is a big stone on the way, because I don't have physical machine and landed OS X just a short time, have many dark hole on it. Face it, solve it, things will be done, I.
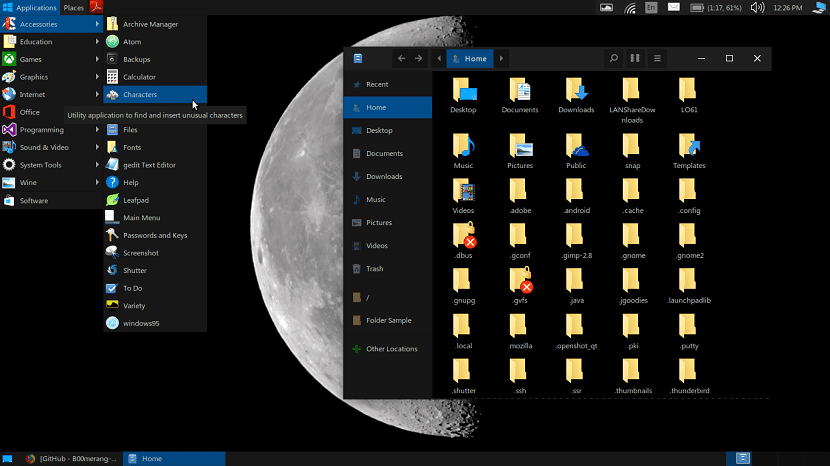
The tutorial demonstrates the use of a button and text label and how to get a handle or pointer to the text label in order to change its text.
Before continuing with the tutorial, install the GTK+ 3 and Glade 3 development tools on your computer.
You may also want to see the very basics of creating a GTK+ 3 program using Glade 3 and C code.
It’s really great that someone has finally ported GTK. I hope someone will create a native Mac theme for GTK, just like Wimp for Windows. @Who is That: No, you won’t be able to run Ximian’s OO.o on OS X (without X11), because OO.o is deeply tied to X11. It uses GTK for the widgets, but most of it doesn’t use GTK. MonoDevelop (also known as Xamarin Studio) is an open-source integrated development environment for Linux, macOS, and Windows. Its primary focus is development of projects that use Mono and.NET frameworks.MonoDevelop integrates features similar to those of NetBeans and Microsoft Visual Studio, such as automatic code completion, source control, a graphical user interface (GUI) and Web designer. GTK+OSX is being developed as the underpinnings for a native Aqua-based Mac OS X port of Film Gimp that's due in the second quarter of the year. 'As soon as we released the Fink-based version of.
Part 5 of GTK 3 Programming with C and Glade Tutorial
This GTK 3 C Code Hello World Tutorial that uses labels and a button can be seen in the following video, updated to use the new Glade UI designer. Refer to the GTK+ 3 reference manual to find the GTK functions used in this tutorial.
GTK 3 C Code Hello World Tutorial Steps
Follow the steps below of this GTK 3 C Code Hello World tutorial to build the application as shown in the previous image. The steps show how to create a new project from template files, design the GUI window in Glade, write the application C code and build the project.
1. Start a New GTK+ 3 Glade C Project

It is easiest to start with a set of GTK+ 3 Glade C programming template files which add a nice structure to the program by separating the C source code files and Glade files into their own folders. The template files include:
- A main.c file containing the function main() in the src folder
- A main glade window called window_main.glade in the glade folder with destory signal callback handler function already connected
- A make file called makefile in the main folder of the project for building the project
- Some skeleton source code in the main.c file that creates the main window of the project using the Glade file
1.1 Create a Set of Template Files
Create a set of template files as described in the article on creating a GTK+ 3 Glade C programming template. Keep this set of template files to use as a base for starting new GTK Glade C projects.
1.2 Start a New Project using the Template Files
Make a copy of the template files folder and rename it to hello.
Open the make file and and change the TARGET name at the top of the file to hello. When the project is built the executable file name will now be named hello.
You should now have a project that looks as follows:
2. Edit the Glade File
Open the glade folder and open window_main.glade in the Glade editor. If the main window does not appear in the Glade editor, click window_main in the right pane.
2.1 Add a Fixed Grid
Although GTK has a method of packing widgets in a window, we will use a fixed grid in this tutorial. Users of MS Visual development tools will be familiar with placing widgets on a grid in a window.
Click the fixed grid icon in the left pane of the Glade window under Containers and then click the main window to place the grid.
Without the fixed grid, buttons and text labels could not be placed.
2.2 Place Text Labels
This project uses two text labels — one to display the hello world text and a second label to display the number of times the button is clicked.
2.2.1 Placing a Label
Click the Label item in the left pane of Glade under the Control and Display section. Click the main window on the grid to place the label. Use the drag / resize tool to move the label to the desired position.
2.2.2 Sizing a Label
Size the label by changing Height request under the Common tab in the right pane of glade to 30.
2.2.3 Change Label ID and Text
In the right pane, with the label selected, click the General tab. Change the ID of the label to lbl_hello in the ID box and the text of the label to … in the Label box.
2.2.4 Add a Second Label
Create a second label with the same height and text as the first label, but give it an ID of lbl_count and place it under the first label.
2.3 Placing and Editing a Button
In the left pane of Glade, click the Button icon under the Control and Display section. Click on the main window grid to place the button. Change the height of the button to 30 in the same place that the height of the text was changed (right pane, Common tab, Height request field).
In the right pane under the General tab, change the button ID to btn_hello and the text of the button to Hello under the Button Content section near the bottom of the right pane. Place the button under the second label.
2.4 Changing the Window Size and Title
Click window_main at the top of the right pane in Glade to select the main window. Under the General tab in the right pane change the window width and height both to 200 in the Default Width and Default Height fields. Change the window title in the Title field to Hello World.
Click the preview snapshot cog icon to see a preview of the window to make sure that the widgets fit in the window properly.
2.5 Button Callback Function Signal
When the button is clicked, it will emit a signal. We need to attach a function to the signal so that the function will be called when the button is clicked.
Click the button in Glade to select it and then click the Signals tab in the right pane of the Glade editor. Click the text under the Handler heading next to clicked to select it and then a second time to start editing it. Type on and then a name suggested by Glade will pop up (on_btn_hello_clicked). Press the down arrow key to select it and then press the Enter key twice to make the change.
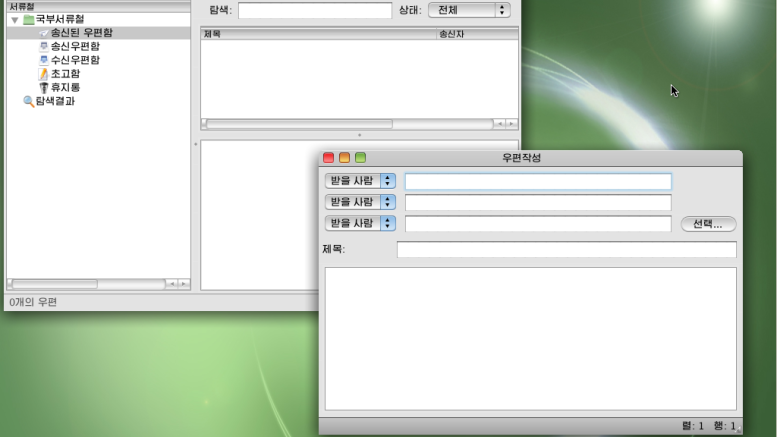
Save the Glade file, we will now edit the C source code.
3. Write the C Code
The final step before building the application is to write the C code for this GTK 3 C Code Hello World Tutorial.
Open the main.c C template file for editing found in the src folder of the project.
We need a pointer to each of the labels in the project so that we can get hold of the labels to change their text. In this simple project, two global pointers are defined at the top of the C file.
After connecting the signals in the code, get pointers to the two labels:

Finally we must add a function that writes text to the first label and increments a count in the second label when it is clicked. This function must have the same name as the signal handler function set for the button in Glade.
The final code should look as follows:
4. Build and Run the Project
Open the template directory in a terminal window and build the project using the make file by entering the make command.
The project can be run by either double-clicking the hello icon after navigating to the project folder using a file manager or from within the terminal window by entering:
The Hello World window should open. Clicking on the Hello button should display the “Hello, world!” text in the first label and increment the count in the second label.
5. GTK 3 C Code Hello World Tutorial Development Environment
Install Gtk For Mac
This tutorial was developed on a Linux Mint 17.3 Cinnamon 64-bit computer with Glade 3.16.1 and GTK+ development library 3.10.8~8+qiana (libgtk-3-dev).
Gtk For Mac
Update 25 October 2016
This tutorial was tested on a Linux Mint 18 Mate 64-bit computer with Glade 3.18.3 and GTK+ development library 3.18.9-1ubuntu3.1 (libgtk-3-dev).
Gtk-mac-bundler
Update 14 October 2019
This tutorial was tested on a Linux Mint 19.2 Cinnamon 64-bit computer with Glade 3.22.1 and GTK+ development library 3.22.30-1ubuntu (libgtk-3-dev). See the video near the top of this article that shows how to build the GUI window using the new Glade which has a different layout.
